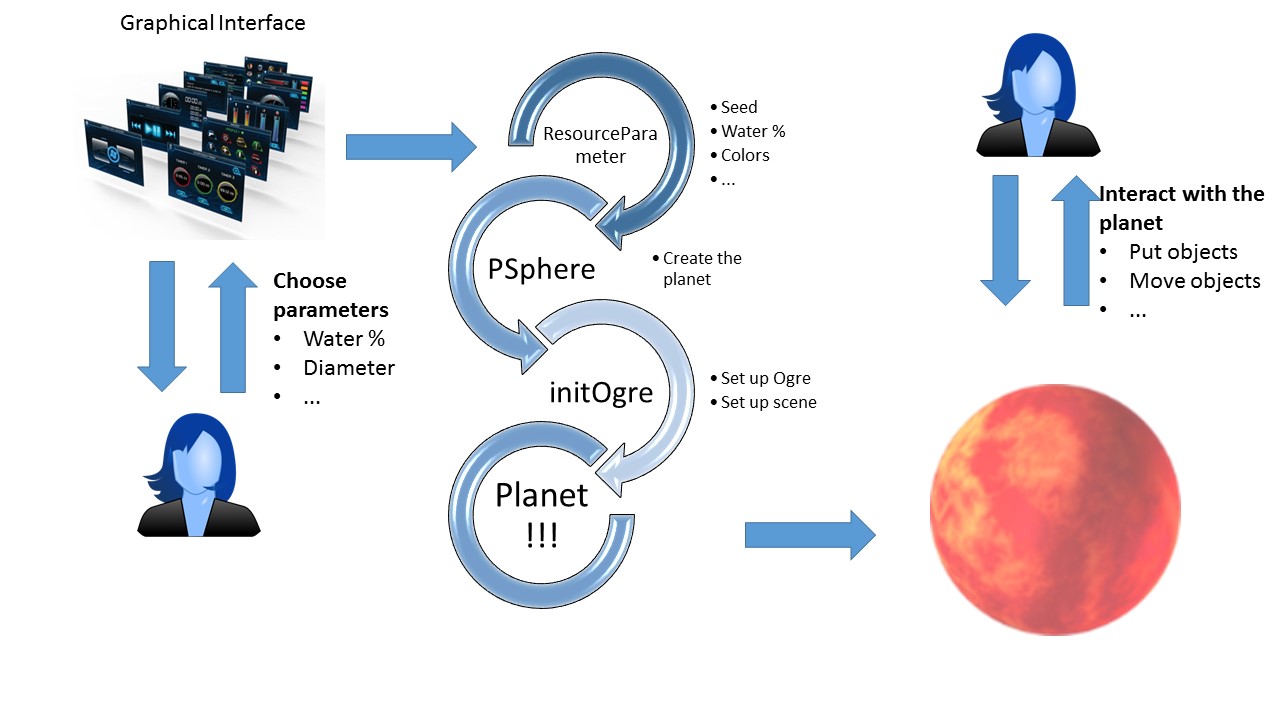
How to start
mySphere = new PSphere(100, 40, 1024, 512, *params);
rendering = new initOgre();
rendering->start();
rendering->setSceneAndRun(mySphere);
delete mySphere;
rendering->cleanup();
delete rendering;
The objects PSphere and ResourceParameter are the only things you need. To set up the Ogre 3D environment (as such the scene and the lights), the class initOgre can be used.
- new PSphere(100, 40, 1024, 512, *params): The object PSphere is created. It's a class representing the planet itself. The planet is set up by the constructor. The first parameter indicates the number of vertices, second grid-resolution, third and fourth texture resolution and fifth is a ResourceParameter object, containing all the information of the planet (for example: water percentage, colors used, radius, etc.)
- new initOgre(): The object initOgre is created.
- start(): It's set up the Ogre 3D environment, such the scene or the lights.
- setSceneAndRun(mySphere): The planet is passed to the Ogre's scene just created, and it starts to show it in the window.
- delete mySphere: The planet is destroid and memory is released.
- rendering->cleanup(): Object relative to the Ogre's scene are destroid.
- delete rendering: Finalize deletion of the object.
Designing the planet
ResourceParameter is the class containing all the information of the planet. This object can be generated by a graphical user interface or it could be even be built inside of a main function. It's a simple object containing variables and getter/setter methods. It has been thought that it can be expanded, if someone need other information to build the planet.
string terrainFirstColor;
string terrainSecondColor;
string waterFirstColor;
string waterSecondColor;
string mountainFirstColor;
string mountainSecondColor;
float waterFraction;
float radius;
int seed;
vector frequency;
vector amplitude;
- terrainFirst/SecondColor- waterFirst/SecondColor - mountainFirst/SecondColor: These indicate the color gradient which charaterizes the enities on the planet. The colors are in hexadecimal format. For example, the first color of the water could represent the deepest point on the sea. The color can be chosen, so the planet can appears in any way possible.
- waterFraction: Percentage of water on the planet (from 0.0 to 100.0). It could be any liquid, since the color is chosen according to the previous parameters.
- radius: Radius of the planet.
- seed: It's a unique number representing the planet. The same seed generate always the same planet.
- frequency: Indicates how much deformed the surface has to be. It is used by the simplex noise algorithm to generates montains and deformation. Higher values permit to have a more deformed suface.
- amplitude: It represents the hichest height of the mountains.
- rendering->cleanup(): Object relative to the Ogre's scene are destroid.
Put objects on the planet
The planet offers a functionality that permits to put and manage objects on the planet. These objects can be virtual, that is objects that can not be seen (such resources like gas, really small things, ghosts or even big things that we don't want to show). Otherwise it's also possible to load already made 3D objects, in .MESH format, with the relative materials (.material files) and textures.
The PHspere class contains a list of ObjectInfo objects, and each of these objects, contain the following informations:
- Position of the object
- Name of the object
- Node to which the object is attached (only in the case of imported 3D objects)
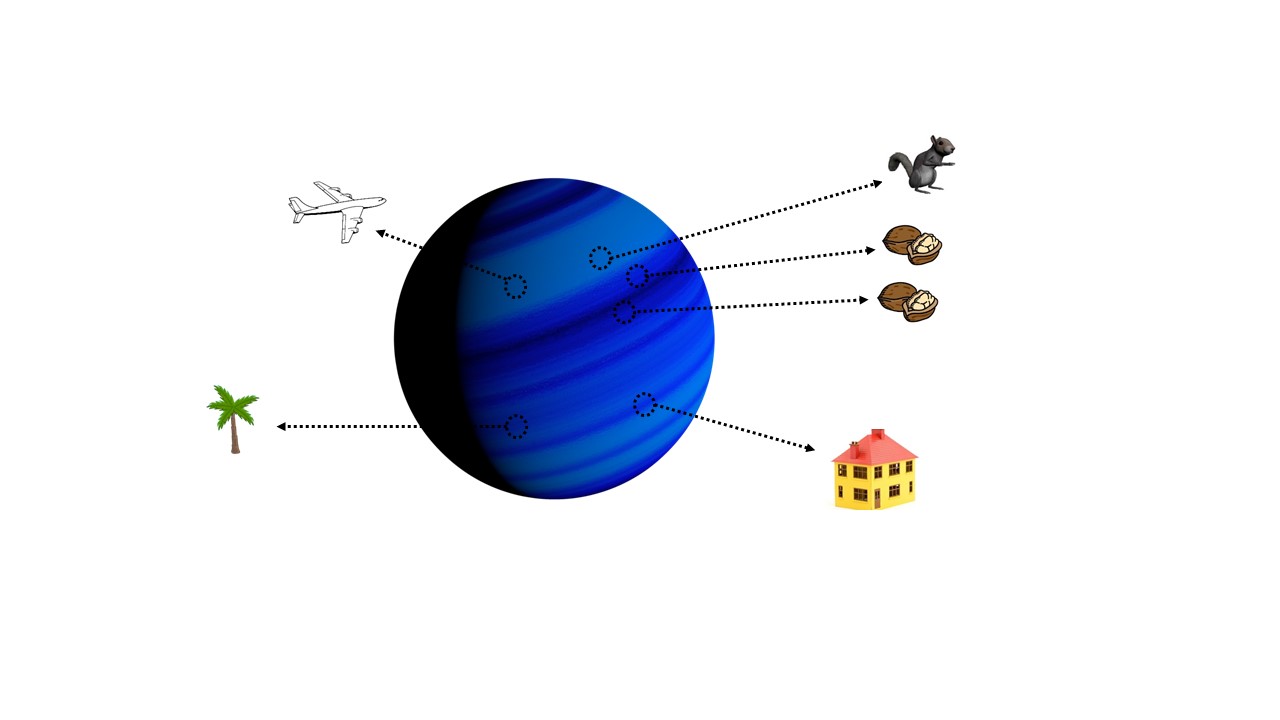
The function which let you put 3D objects (.MESH files) on the planet is attachMesh() of the class PSphere. You can check in any moment if an object with the same name has already been put on the planet with the function checkIfObjectIsIn().
Accessibility
The planet provides a functionality to know where the liquid part (usually water) is. This is usefull if someone want to interact with the planet, and need to know where the water is, for example to move objects on the surface (animals, vehicles etc.). The accessibility system is made through a binary grid, which says where the water is.
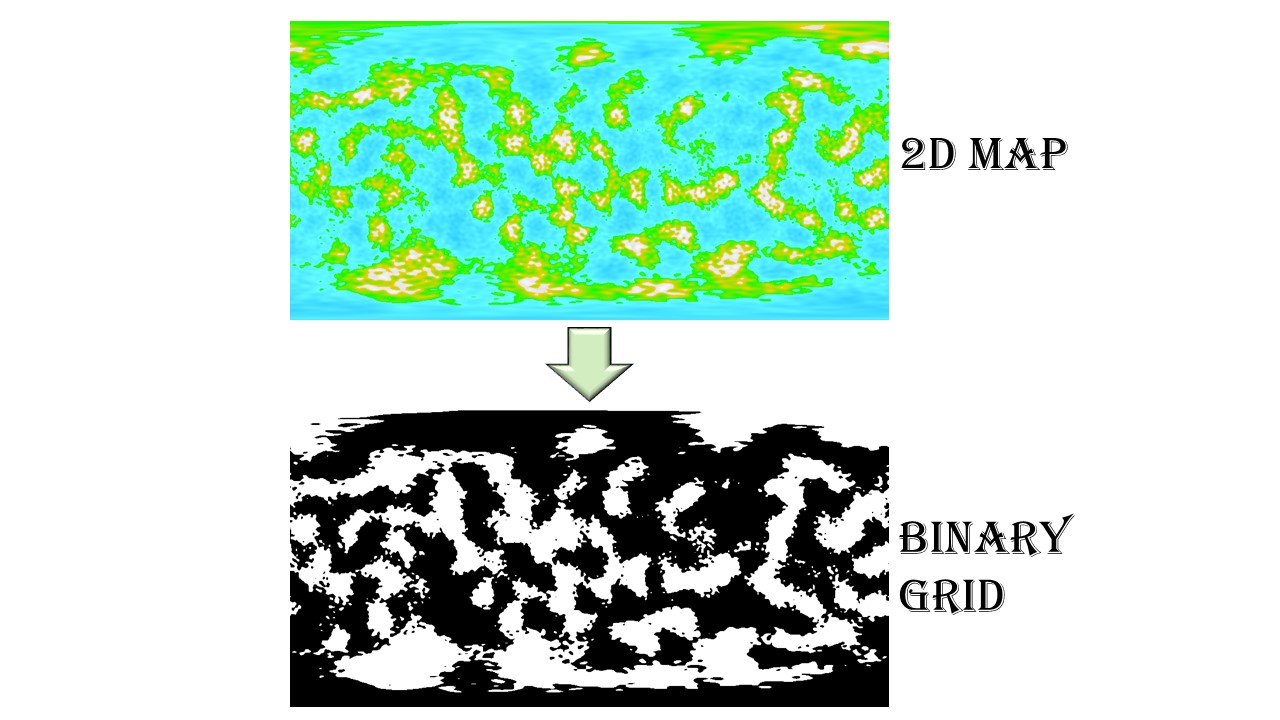
As in the previous image, the grid can be thought black and white, where the black part represents the water. To ask the planet where the water it has been provided the function checkAccessibility() where you can specify the position, and it returns a boolean value which indicates if there's water or not. Not only that ! The previous function says also if in a certain position there's already another object, so there's no possibility to put another one. So the returned value is true if there are no objects in the specified position.
Graphical User Interface
This project provides also a GUI which gives a user-frindly way to specify planet's parameters. This GUI has been created using QT. As the project is developed at the moment, the GUI starts immediately before generating the planet. So the code which starts the planet's rendering it's inside of the GUI main code. It can simply moved to another position if the use of the GUI is not desired. How the GUI works can be seen in the usage page.